Hello,
I am trying to run an Azure Function as an API between a React Native Application and a Microsoft SQL database. I want users to be able to log in with Microsoft credentials and access data. I have an access token from an app registration (named Bear Necessities AD) in Entra ID using the following code.
const getSession = async () => {
const cv = await Crypto.digestStringAsync(
Crypto.CryptoDigestAlgorithm.SHA256,
Math.random().toString(36).substr(2) + Date.now().toString(36)
);
const d = await AuthSession.fetchDiscoveryAsync(domain);
const cc = await base64URLEncode(cv);
const authRequestOptions: AuthSession.AuthRequestConfig = {
prompt: AuthSession.Prompt.Login,
responseType: AuthSession.ResponseType.Code,
scopes: scopes,
usePKCE: true,
clientId: ADConfig.applicationClientID,
//clientSecret: ADConfig.secretValue,
redirectUri: __DEV__ ? redirectUrl : redirectUrl + "example",
state: ADConfig.state,
codeChallenge,
codeChallengeMethod: AuthSession.CodeChallengeMethod.S256,
};
const authRequest = new AuthSession.AuthRequest(authRequestOptions);
$authRequest(authRequest);
$discovery(d);
$codeChallenge(cc);
};
const getCodeExchange = async () => {
const tokenResult = await AuthSession.exchangeCodeAsync(
{
code: authorizeResult.params.code,
clientId: ADConfig.applicationClientID,
redirectUri: __DEV__ ? redirectUrl : redirectUrl + "example",
extraParams: {
code_verifier: authRequest.codeVerifier,
grant_type: "authorization_code",
},
},
discovery
);
const { accessToken, refreshToken, issuedAt, expiresIn } = tokenResult;
$token(tokenResult);
console.log(accessToken);
console.log(refreshToken);
console.log(issuedAt);
console.log(expiresIn);
};
I have added Microsoft as the identity provider for the function app, using the same application registration.
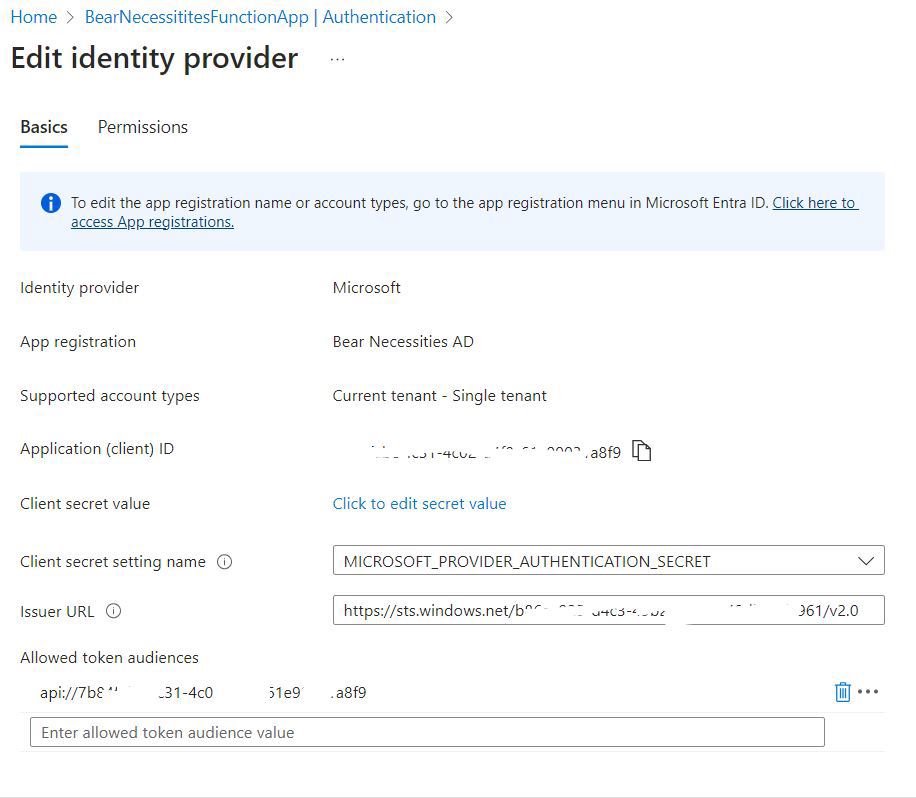
Here is my attempt to expose the api:
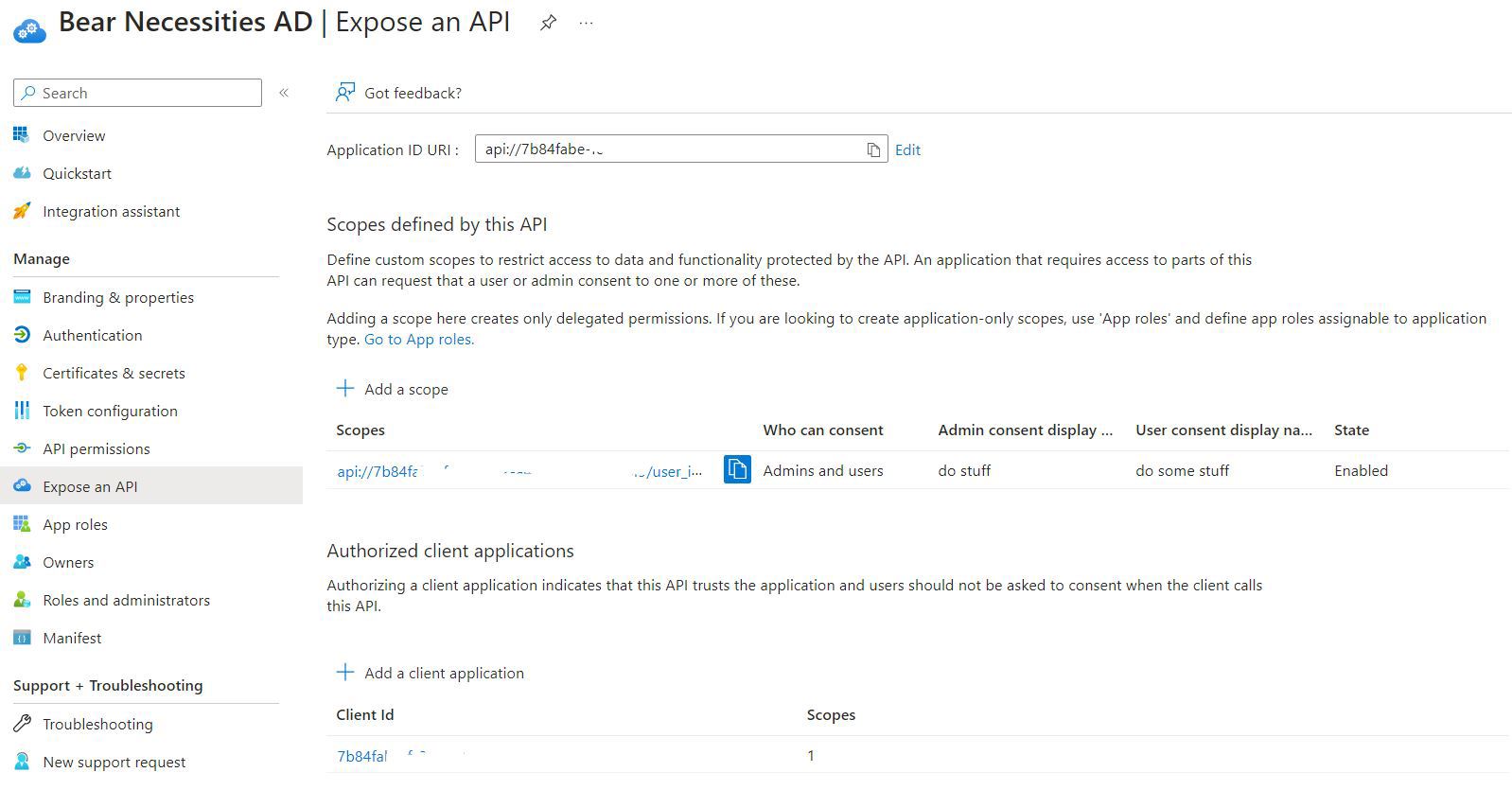
Here is the code I use to call the function:
const callAzureFunction = async () => {
const functionUrl =
"https://bearnecessititesfunctionapp.azurewebsites.net/api/HelloWorld?code=XXXXXXX==";
try {
const response = await fetch(functionUrl, {
method: "POST", // Or 'POST', 'PUT', etc., depending on your Azure Function configuration
headers: {
Authorization: `Bearer ${token.accessToken}`,
"Content-Type": "application/json",
},
body: JSON.stringify({
name: "Test",
}),
});
if (response.ok) {
const data = await response.json();
console.log("Response from Azure Function:", data);
// Process the data received from the Azure Function
console.log(data);
} else {
console.error("Error calling Azure Function:", response.status);
// Handle error responses
}
} catch (error) {
console.error("Error:", error);
// Handle any network errors or exceptions
}
};
I can call the function when authentication is disabled, but I get the set 401 error when it is enabled. Am I able to authenticate using the access token I already have? If so, what could be wrong here?
Thank you for your time!