I have a requirement to upload csv files into onedrive personal using python in non-ineractive way. I have created an app registration in my Azure tenant with below API permissions
Type:
Accounts in any organizational directory (Any Microsoft Entra ID tenant - Multitenant) and personal Microsoft accounts (e.g. Skype, Xbox)"
API Permissions:
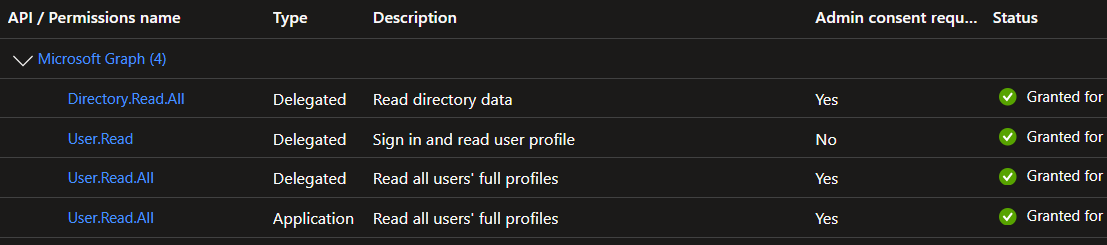
My Code:
import requests
# Replace with your details
client_id = 'xxxxx'
client_secret = 'xxxx'
tenant_id = '052c8b5b-xxxx'
filename = 'C:/test.csv'
onedrive_folder = 'CloudOnly/test'
user_id = 'xxxx-e7d1-44dc-a846-5exxxx'
# Get the access token
url = f'https://login.microsoftonline.com/{tenant_id}/oauth2/v2.0/token'
data = {
'grant_type': 'client_credentials',
'client_id': client_id,
'client_secret': client_secret,
'scope': 'https://graph.microsoft.com/.default'
}
response = requests.post(url, data=data)
token = response.json().get('access_token')
# Upload the file to OneDrive
headers = {
'Authorization': f'Bearer {token}',
'Content-Type': 'application/octet-stream'
}
file_content = open(filename, 'rb').read()
upload_url = f'https://graph.microsoft.com/v1.0/users/{user_id}/drive/root:/{onedrive_folder}/{filename.split("/")[-1]}:/content'
upload_response = requests.put(upload_url, headers=headers, data=file_content)
if upload_response.status_code == 201:
print('File uploaded successfully!')
else:
print('Error uploading file:', upload_response.json())
Error message:
Error uploading file: {'error': {'code': 'BadRequest', 'message': 'Tenant does not have a SPO license.', 'innerError': {'date': '2025-01-14T02:15:47', 'request-id': 'cf70193e-1723-44db-9f5e-xxxxx', 'client-request-id': 'cf70193e-1723-44db-9f5e-xxxx'}}}
How to solve this ? Please note, I need to use a non-interactive way as this has to be setup on an ubuntu server to run the script on daily basis and upload the CSV files to personal onedrive.