Metodo ID2D1RenderTarget::FillRoundedRectangle(constD2D1_ROUNDED_RECT&,ID2D1Brush*) (d2d1.h)
Disegna l'interno del rettangolo arrotondato specificato.
Sintassi
void FillRoundedRectangle(
const D2D1_ROUNDED_RECT & roundedRect,
[in] ID2D1Brush *brush
);
Parametri
roundedRect
Tipo: [in] const D2D1_ROUNDED_RECT &
Dimensioni del rettangolo arrotondato da disegnare, in pixel indipendenti dal dispositivo.
[in] brush
Tipo: [in] ID2D1Brush*
Pennello utilizzato per disegnare l'interno del rettangolo arrotondato.
Valore restituito
nessuno
Osservazioni
Questo metodo non restituisce un codice di errore in caso di errore. Per determinare se un'operazione di disegno (ad esempio FillRoundedRectangle) non è riuscita, controllare il risultato restituito dai metodi ID2D1RenderTarget::EndDraw o ID2D1RenderTarget::Flush .
Esempio
Nell'esempio seguente vengono utilizzati i metodi DrawRoundedRectangle e FillRoundedRectangle per strutturare e riempire un rettangolo arrotondato. In questo esempio viene generato l'output illustrato nella figura seguente.
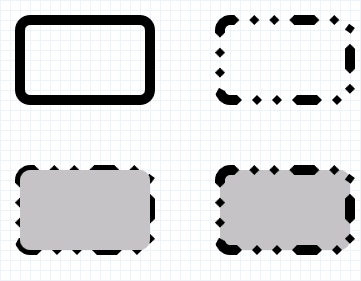
// Called whenever the application needs to display the client
// window.
HRESULT DrawAndFillRoundedRectangleExample::OnRender()
{
HRESULT hr;
// Create the render target and brushes if they
// don't already exists.
hr = CreateDeviceResources();
if (SUCCEEDED(hr))
{
// Retrieve the size of the render target.
D2D1_SIZE_F renderTargetSize = m_pRenderTarget->GetSize();
m_pRenderTarget->BeginDraw();
m_pRenderTarget->SetTransform(D2D1::Matrix3x2F::Identity());
m_pRenderTarget->Clear(D2D1::ColorF(D2D1::ColorF::White));
// Paint a grid background.
m_pRenderTarget->FillRectangle(
D2D1::RectF(0.0f, 0.0f, renderTargetSize.width, renderTargetSize.height),
m_pGridPatternBitmapBrush
);
// Define a rounded rectangle.
D2D1_ROUNDED_RECT roundedRect = D2D1::RoundedRect(
D2D1::RectF(20.f, 20.f, 150.f, 100.f),
10.f,
10.f
);
// Draw the rectangle.
m_pRenderTarget->DrawRoundedRectangle(roundedRect, m_pBlackBrush, 10.f);
// Apply a translation transform.
m_pRenderTarget->SetTransform(D2D1::Matrix3x2F::Translation(200.f, 0.f));
// Draw the rounded rectangle again, this time with a dashed stroke.
m_pRenderTarget->DrawRoundedRectangle(roundedRect, m_pBlackBrush, 10.f, m_pStrokeStyle);
// Apply another translation transform.
m_pRenderTarget->SetTransform(D2D1::Matrix3x2F::Translation(0.f, 150.f));
// Draw, then fill the rounded rectangle.
m_pRenderTarget->DrawRoundedRectangle(roundedRect, m_pBlackBrush, 10.f, m_pStrokeStyle);
m_pRenderTarget->FillRoundedRectangle(roundedRect, m_pSilverBrush);
// Apply another translation transform.
m_pRenderTarget->SetTransform(D2D1::Matrix3x2F::Translation(200.f, 150.f));
// Fill, then draw the rounded rectangle.
m_pRenderTarget->FillRoundedRectangle(roundedRect, m_pSilverBrush);
m_pRenderTarget->DrawRoundedRectangle(roundedRect, m_pBlackBrush, 10.f, m_pStrokeStyle);
hr = m_pRenderTarget->EndDraw();
if (hr == D2DERR_RECREATE_TARGET)
{
hr = S_OK;
DiscardDeviceResources();
}
}
return hr;
}
Requisiti
Requisito | Valore |
---|---|
Client minimo supportato | Windows 7, Windows Vista con SP2 e Aggiornamento della piattaforma per Windows Vista [app desktop | App UWP] |
Server minimo supportato | Windows Server 2008 R2, Windows Server 2008 con SP2 e Platform Update per Windows Server 2008 [app desktop | App UWP] |
Piattaforma di destinazione | Windows |
Intestazione | d2d1.h |
Libreria | D2d1.lib |
DLL | D2d1.dll |